Rescuing Important Emails from the Junk Folder with Microsoft Graph API
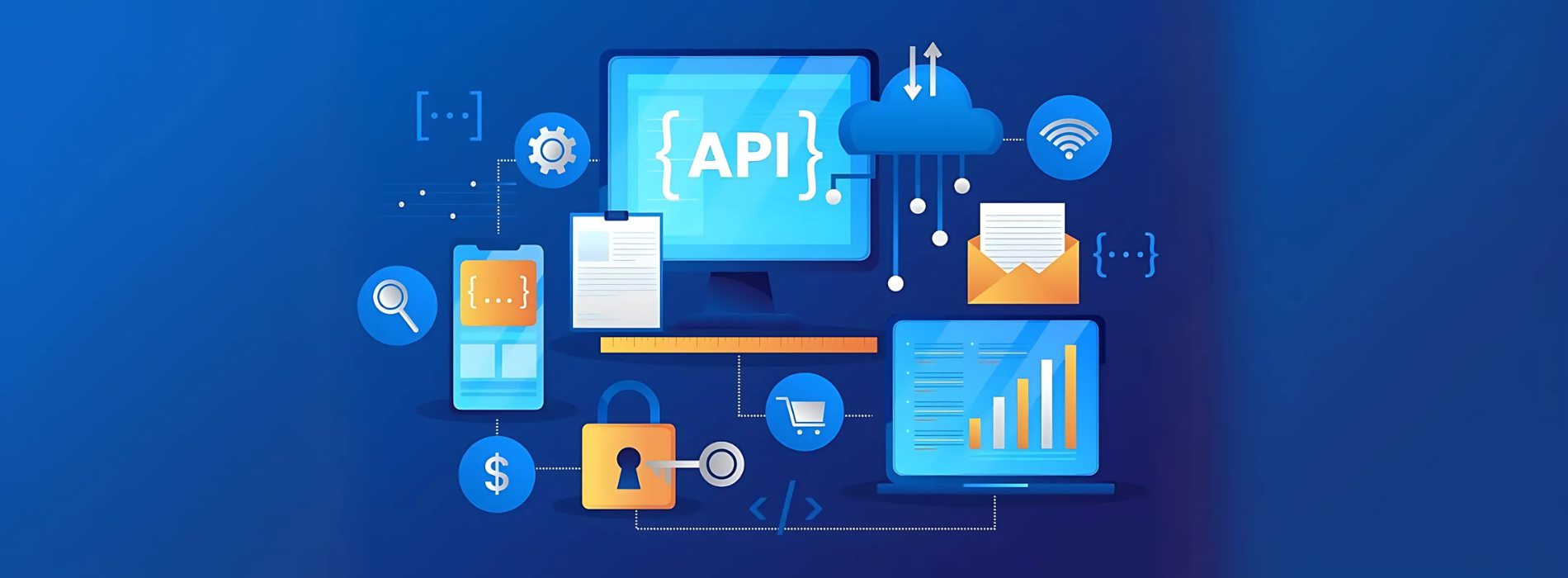
Introduction: Because, Of Course, It Had to Be the CEO
Let me paint you a picture: we were managing the Microsoft 365 environment for one of our long-time customers, let’s call them Company A. We had everything running smoothly—mailboxes organized, Teams working like a charm, and security policies in place. You know, the usual IT perfection (or at least close enough!). Then, out of nowhere, Company A gets bought by Company B—another company that was also using Microsoft 365 but on a completely different tenant. I mean, what’s better than managing one tenant? Managing two, of course! 🙄
The plan was simple: assess the current environment, plan the migration, and move over a few hundred—okay, maybe a few thousand—users from Tenant A to Tenant B. Easy, right? Well, it would have been if the CEO of Company B (now CEO of both companies) hadn’t decided to send a heartfelt, company-wide welcome email to all employees from Company A. You know, one of those, “Welcome to the family, let’s make magic happen together” emails.
Sounds nice, right? Except that for some reason, this email didn’t land in everyone’s inbox. Oh no, it decided to take a detour straight into the junk folder of several employees in Tenant A. And of course, it couldn’t be just anyone. Nope—it’s always the CEO, CFO, or some other high-level executive who faces this kind of issue. Why is it always the top brass? I’m convinced it’s the universe’s way of keeping us humble
So there we were, tasked with quietly and efficiently moving the CEO’s email out of the junk folder and into the inbox—without raising any eyebrows, of course. No one needs to know that the new CEO’s warm welcome was rejected by the company’s spam filter
That’s where the Microsoft Graph API comes in to save the day (and our sanity). In this blog, I’m going to walk you through how we used the Graph API to find those misplaced emails and move them to the inbox, all without anyone even noticing. You’ll get code samples, tips, and maybe a few laughs along the way—because, let’s be honest, if we can’t laugh at our IT woes, what else can we do?
Stick around, and I’ll show you how to become the email-moving ninja your CEO desperately needs. Ready? Let’s dive in!
What You’ll Need to Become an Email-Rescuing Ninja
Alright, let’s get into the nitty-gritty of how we’re going to rescue those poor, misplaced emails from the junk folder using the Microsoft Graph API. Before we start flipping bits and bytes, here’s what we’ll be doing (and don’t worry, I’ll walk you through it step by step—funny analogies included).
Step 1: Authenticating with the Graph API (Because We Need the Keys to the Castle Before We Can Move Anything Around)
Before we can start shuffling emails from the junk folder to the inbox, we need permission. Think of it like trying to get into a fancy club—you need to show your VIP pass at the door. In our case, that VIP pass is the OAuth2 access token, which lets us call the Microsoft Graph API to interact with users’ mailboxes.
In this step, we’ll be:
- Setting up app registration in Azure AD (because no API wants to talk to just anyone).
- Getting the appropriate permissions to read and write emails using the Mail. ReadWrite scope.
- Generating our access token, which is like getting the master key to every user’s mailbox. (Don’t worry, we’ll be responsible with this power. It’s not like we’re looking for juicy gossip or anything.)
Step 2: Searching for Those Sneaky Emails in the Junk Folder
Once we’ve got our access token (a.k.a. the keys to the castle), it’s time to go email hunting. The good news is, the Graph API is like a professional detective—it’ll help us track down those misplaced CEO emails that thought they could hide in the junk folder.
We’ll use the API to:
- Search through the JunkEmail folder for emails with specific subjects, senders, or time frames (in this case, our poor CEO’s welcome message).
- Get the email IDs of the junked messages so we know exactly which ones to move.
Think of it like finding that one sock that always goes missing after laundry day. You know it’s there somewhere, hiding in plain sight.
Step 3: Moving Emails to the Inbox—Where They Belong (Like Putting Socks in the SockDrawer After Laundry Day)
Now that we’ve found the elusive CEO email in the junk folder, it’s time to move it where it rightfully belongs—the inbox. This is the digital equivalent of putting socks back in the sock drawer after laundry day. It’s a simple act, but one that makes all the difference in avoiding chaos. 😅
In this step, we’ll:
- Use the Graph API’s move endpoint to relocate the emails from the junk folder to the inbox.
- Make sure everything is neatly organized in its proper place—no more important
emails getting flagged as junk
Step 4: Doing All This Without Tipping Off the Users (Stealth Mode: Activated!)
Finally, we’ve got to make sure all this happens without anyone noticing. No one needs to know that their brand-new CEO’s heartfelt welcome email was considered digital garbage by the spam filter. We’ll move the emails in stealth mode—silent, efficient, and completely under the radar
In this step, we’ll:
- Ensure the users aren’t alerted by unnecessary notifications.
- Keep everything quiet, like a ninja slipping into the shadows after a job well done.
Because the last thing you want is for someone to ask, “Hey, why did the CEO’s email land in junk?”
Step 1: Authenticating with the Graph API (Because No Ninja Gets into the Castle Without the Right Keys)
Alright, warriors, the first step of our mission is to secure access to the Graph API—this is your golden ticket to all the inbox-saving power. But, like any good ninja, we don’t just barge in through the front door. We need to sneak in the right way by grabbing an OAuth2 token that’ll let us call the Graph API like pros. Ready to get your key to the castle? Let’s break it down:
Step 1.1: Registering Your App in Azure AD (The Secret Entrance)
To get started, you need to register your app in Azure Active Directory. This is where we create a stealthy identity for our app, which we’ll use to request the magical token that gives us access.
- Head over to the Azure Portal and sign in.
- In the left-hand menu, click Azure Active Directory.
- Go to App Registrations and hit New Registration.
- Give your app a name (something cool like “NinjaEmailMover”).
- Under Supported account types, select Accounts in this organizational directory only (if you’re only working within your organization).
- For the Redirect URI, choose Public client/native and enter https://login.microsoftonline.com/common/oauth2/nativeclient.
- Click Register, and boom—you’ve just created the app that will let you perform your ninja magic
Step 1.2: Granting Permissions to the App (Power Up)
Now that we’ve registered the app, we need to give it the right
permissions
to read and move emails. Because without the right permissions, our ninja tools are pretty much useless.
- In your newly created app, go to API Permissions.
- Click Add a permission, then choose Microsoft Graph.
- Select Delegated Permissions and check the following:
-
- > Mail.ReadWrite (Allows your app to read and move emails)
- > User.Read (This one’s default, and it’s just to read basic user profile info)
-
- Once you’ve added the permissions, click Grant admin consent to give your app the green light to actually use them.
Now your app has the power it needs to read and move emails. Pretty cool, right? 🔥
Step 1.3: Creating a Client Secret (Your Ninja Tool)
Next up, we need to create a Client Secret. This is like your app’s katana—it’ll let you authenticate and request access tokens when you call the Graph API.
- Go to Certificates & Secrets in your app’s settings.
- Click New client secret.
- Give it a description (like “NinjaSecret”) and choose an expiration time.
- Click Add.
- Important: Copy the secret value and store it somewhere safe (not on a Post-it note!). You’ll need it to authenticate your app, and you won’t be able to see it again after you leave this page.
Step 1.4: Store That Token Securely (Guard It Like a True Ninja)
Your token is your pass to the API, and just like any secret tool in your ninja arsenal, you need to protect it. This token is typically valid for 60 minutes, so make sure you refresh
it before it expires.
What This Script Does?
What This Script Does?
- Authenticate: It first grabs an OAuth2 access token so we can communicate with the Microsoft Graph API.
- Search the Junk Folder: For each user, the script will search the Junk Email folder for emails matching a specific subject.
- Move Emails: If the email is found, it will be copied (moved) to the user’s inbox.
- Log Progress: We’ll get live feedback from the script on whether emails were found and moved successfully or not.
Step 1: Authentication (Because We Need Permission to Move Stuff)
Before we start rummaging through users’ junk folders, we need to authenticate with the Graph API. This is done using OAuth2, and the script will request an access token by passing in the ClientID, TenantID, and ClientSecret of our Azure AD app.
Here’s the function that handles this for us:
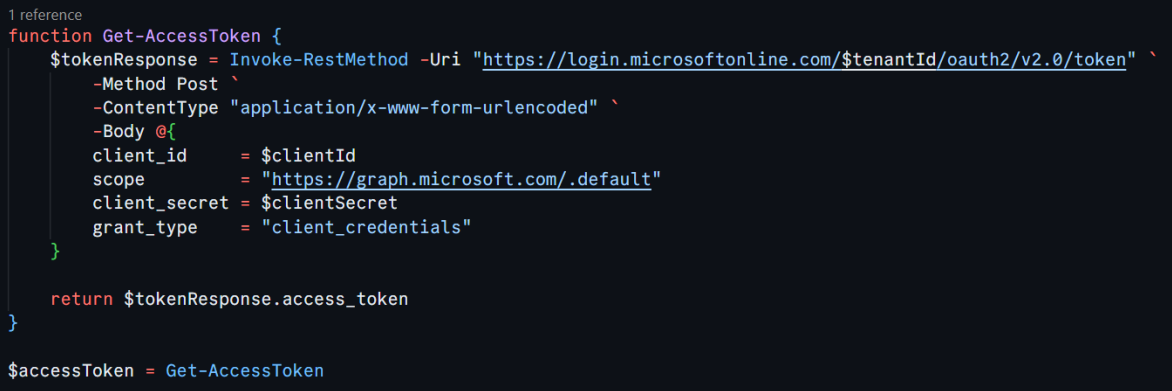
This function sends a request to Azure AD, asking for a token that gives us permission to access users’ mailboxes. You’ll need to replace , , and with your actual values from your Azure AD app registration. This token is our “all-access pass” to the Graph API. Fun fact: Getting this token feels like having the master key to the building…except this key only opens inboxes and junk folders. 🗝️
Step 2: Reading User Emails from a File (Bulk Operations for the Win)
To avoid manually specifying each user, this script reads a list of users from a text file. Each email in the file will be processed in turn. Here’s how we grab that list of users:

Each user’s email address should be listed on a new line in the text file. The script will iterate over this list and handle junk email detection for each user. It’s a nice bulk operation—no need to handle one user at a time.
Step 3: Searching for Emails in the Junk Folder (Ninja Radar On)
Now, for each user in our list, we’ll search their JunkEmail folder for any messages that match the specified subject. We’re using the Microsoft Graph API to do this

This part of the script constructs the Graph API URL that targets the JunkEmail folder for a particular user ($userEmail). The ?$filter=subject eq ‘$emailSubject’ part filters the emails to only those matching the subject you specify.
It’s like being a ninja detective, scanning for emails that don’t belong in the shadows of the junk folder. 🥷📧
Step 4: Moving the Email to the Inbox (Time to Strike)
Once we’ve located the email in the junk folder, we need to move it to the inbox where it belongs. Here’s how we do that:
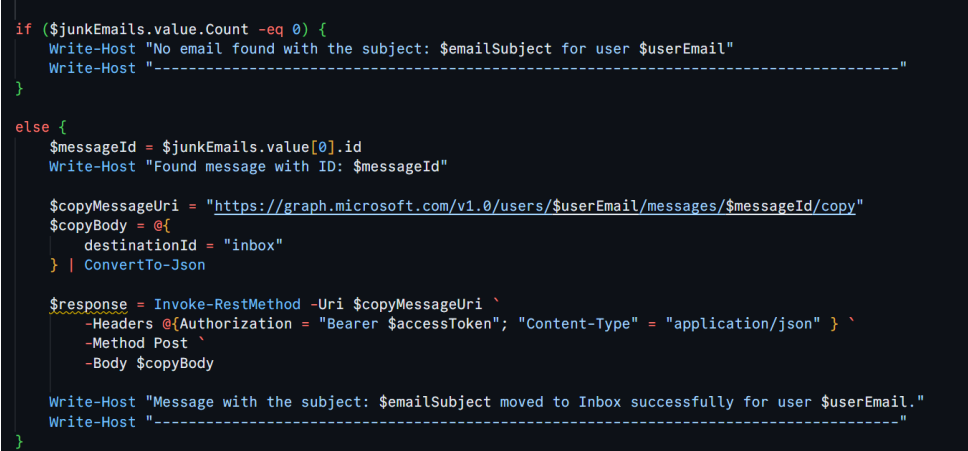
Here’s what happens in this block:
- First, we check if any matching emails were found in the junk folder ($junkEmails.value.Count -eq 0).
- If no email is found, the script logs a message and moves on to the next user.
- If an email is found, we extract the message ID and construct the API call to move (copy) it to the inbox.
- The destinationId = “inbox” specifies where the email will be moved.
Step 5: Logging the Results (Because Feedback is Key)
The script gives you live feedback about whether it found an email and successfully moved it. This way, you can monitor what’s happening and make sure the operation runs smoothly. You’ll know exactly what’s going on, and you can intervene if something looks off.
Wrapping It Up: Ninja Level 100 Achieved!
And there you have it! With just a few lines of PowerShell and the power of the Microsoft Graph API, you’ve become a master of email movement, whisking important messages out of the junk folder and into the inbox—all without breaking a sweat.
This script is especially handy if you’re managing multiple users and don’t want to dig through each junk folder manually. Now, you can let PowerShell and the Graph API do the heavy lifting while you take the credit for saving the day.
So next time a CEO’s email ends up in the junk folder, you’ll be ready. Just don’t forget to add this to your IT ninja toolbox! 🥷✨
Have any questions or issues? Drop them in the comments below, and let’s troubleshoot together!
No Comments